Props란?
props는 변하지 않는 데이터이다. props의 데이터 흐름은 상위 Component에서 하위 Component로 이동한다고 볼 수 있다. 아래 코드를 예시로 보자.
import React, { Component } from 'react';
import {View, Text} from 'react-native';
class PropsText extends Component {
constructor(props){
super(props);
}
render(){
return(<Text>{this.props.text}</Text>);
}
}
const App = () => {
return (
<View
style={{
flex: 1,
flexDirection: 'column',
justifyContent: 'space-evenly',
}}>
<PropsText text = 'first'></PropsText>
<PropsText text = 'second'></PropsText>
<PropsText text = 'third'></PropsText>
</View>
);
};
export default App;
PropsText라는 임의의 class를 만들었다. 전달받은 props를 <Text>에 적용시켜 render 하는 간단한 Component이다.
App에서 <PropsText>라는 Compnent를 세번 render 시킨다.
각각의 <PropsText>에 'first', 'second', 'third'라는 props를 전달했다. 그 후, <PropsText>는 전달받은 props를 적용시켜 render 한다.
다음은 출력 화면이다.
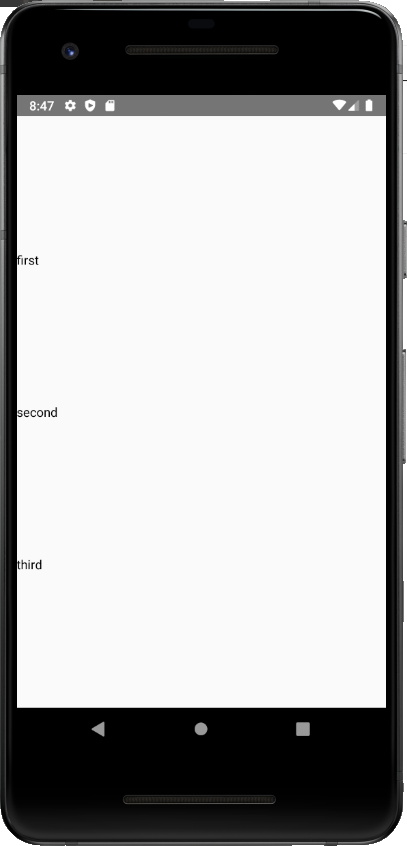
state란?
state는 변할 수 있는 값을 다룰 때 사용한다. 자주 변경되는 Component, Server에서 데이터를 받아와 render 하는 component 같은 경우에 자주 사용한다.
다음은 예시 코드이다.
import React, {Component} from 'react';
import {View, Text, TextInput} from 'react-native';
class StateText extends Component {
constructor(props) {
super(props);
this.state = {
text: ' ',
}
}
render() {
return (
<View style={{flexDirection: 'column', justifyContent: 'space-evenly'}}>
<TextInput
placeholder="입력하세요"
onChangeText={(text) => this.setState({text: text})}
/>
<Text>{this.state.text}</Text>
</View>
);
}
}
const App = () => {
return (
<View
style={{
flex: 1,
flexDirection: 'column',
justifyContent: 'center',
alignItems: 'center',
}}>
<StateText />
</View>
);
};
export default App;
StateText라는 Component를 만들었다. <TextInput>과 <Text> 태그를 나열한 Component이며, <TextInput>에 입력받은 내용을 <Text>에 적용시켜 출력하는 Component이다.
<TextInput>에 입력이 될 때, onChangeText에 설정한 함수가 동작한다. 화살표 함수를 사용하여 setState라는 함수를 작동시켰다. setState는 해당하는 Component의 state값을 변경시키는 함수이다. state는 함부로 변경시키면 안 되기 때문에 무조건 setState함수를 사용하여 변경해야 한다.
다음은 출력 화면이다.
![]() |
![]() |
Props란?
props는 변하지 않는 데이터이다. props의 데이터 흐름은 상위 Component에서 하위 Component로 이동한다고 볼 수 있다. 아래 코드를 예시로 보자.
import React, { Component } from 'react';
import {View, Text} from 'react-native';
class PropsText extends Component {
constructor(props){
super(props);
}
render(){
return(<Text>{this.props.text}</Text>);
}
}
const App = () => {
return (
<View
style={{
flex: 1,
flexDirection: 'column',
justifyContent: 'space-evenly',
}}>
<PropsText text = 'first'></PropsText>
<PropsText text = 'second'></PropsText>
<PropsText text = 'third'></PropsText>
</View>
);
};
export default App;
PropsText라는 임의의 class를 만들었다. 전달받은 props를 <Text>에 적용시켜 render 하는 간단한 Component이다.
App에서 <PropsText>라는 Compnent를 세번 render 시킨다.
각각의 <PropsText>에 'first', 'second', 'third'라는 props를 전달했다. 그 후, <PropsText>는 전달받은 props를 적용시켜 render 한다.
다음은 출력 화면이다.
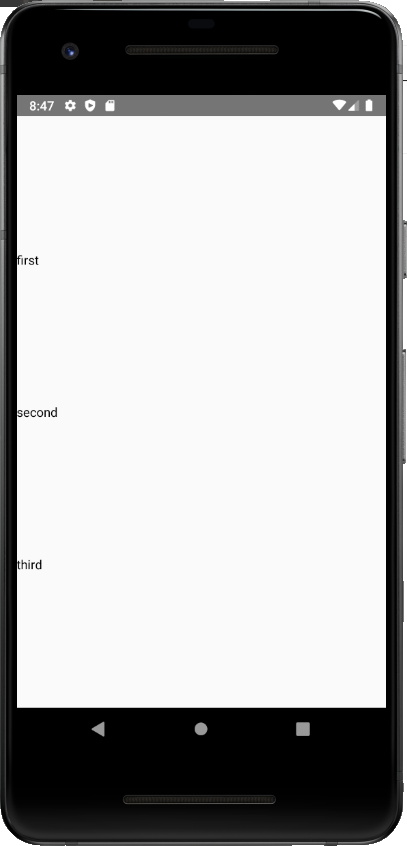
state란?
state는 변할 수 있는 값을 다룰 때 사용한다. 자주 변경되는 Component, Server에서 데이터를 받아와 render 하는 component 같은 경우에 자주 사용한다.
다음은 예시 코드이다.
import React, {Component} from 'react';
import {View, Text, TextInput} from 'react-native';
class StateText extends Component {
constructor(props) {
super(props);
this.state = {
text: ' ',
}
}
render() {
return (
<View style={{flexDirection: 'column', justifyContent: 'space-evenly'}}>
<TextInput
placeholder="입력하세요"
onChangeText={(text) => this.setState({text: text})}
/>
<Text>{this.state.text}</Text>
</View>
);
}
}
const App = () => {
return (
<View
style={{
flex: 1,
flexDirection: 'column',
justifyContent: 'center',
alignItems: 'center',
}}>
<StateText />
</View>
);
};
export default App;
StateText라는 Component를 만들었다. <TextInput>과 <Text> 태그를 나열한 Component이며, <TextInput>에 입력받은 내용을 <Text>에 적용시켜 출력하는 Component이다.
<TextInput>에 입력이 될 때, onChangeText에 설정한 함수가 동작한다. 화살표 함수를 사용하여 setState라는 함수를 작동시켰다. setState는 해당하는 Component의 state값을 변경시키는 함수이다. state는 함부로 변경시키면 안 되기 때문에 무조건 setState함수를 사용하여 변경해야 한다.
다음은 출력 화면이다.
![]() |
![]() |