Get Weather
이전 포스트에서 위치를 받아와 위도, 경도를 알아내 log를 찍어보았다.
이제는 위도, 경도를 이용하여 해당 지역에 날씨를 받아오는 일을 하면 프로젝트의 전반적인 부분을 다 마치게 된다.
날씨를 받아오는 방법은 아래의 api를 이용한다.
Сurrent weather and forecast - OpenWeatherMap
Dashboard and Agro API We provide satellite imagery, weather data and other agricultural services that are based on geodata. By using Agro API , you can easily power your solution based on this information. Dashboard is a visual service where you can easy
openweathermap.org
회원 가입을 하면 api key를 부여받을 수 있다.
https://openweathermap.org/current
Current weather data - OpenWeatherMap
Access current weather data for any location on Earth including over 200,000 cities! Current weather is frequently updated based on global models and data from more than 40,000 weather stations. Data is available in JSON, XML, or HTML format. We provide 40
openweathermap.org
이 페이지에 나오는
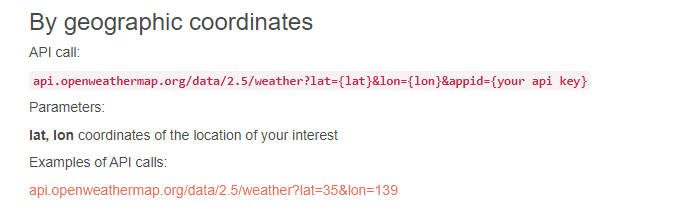
위도, 경도 좌표로 날씨를 받아오는 api를 참고하여 이전 포스트에 받은 위도, 경도를 입력하면 완료된다.
다음은 수정된 App.js이다.
import React from 'react';
import { StyleSheet, Text, View } from 'react-native';
import Loading from './Loading';
import * as Location from 'expo-location';
import * as axios from 'axios';
const API_key = 'e096ae46f45b79ac3723946f3382abb6';
export default class App extends React.Component{
constructor(props){
super(props);
}
state = {
errorMsg: ' ',
isLoading: true,
}
getLocation = async() =>{
const status = await Location.requestPermissionsAsync();
if (status !== 'granted') {
this.setState({errorMsg : 'Permission to access location was denied'});
console.log(this.state.errorMsg);
}
const {
coords: {latitude, longitude}
} = await Location.getCurrentPositionAsync();
this.getWeather(latitude, longitude);
}
getWeather = async(latitude, longitude) => {
const data = await axios.get(
`http://api.openweathermap.org/data/2.5/weather?lat=${latitude}&lon=${longitude}&appid=${API_key}`
);
console.log(data);
}
componentDidMount(){
this.getLocation();
}
render(){
return <Loading/>;
}
}
Location.getCurrentPositionAsync()를 통해서 위치 정보를 얻어왔다 그 얻어온 정보 중, coord라는 객체 안에 들어있는 latitude, longitude를 받아온다.
그리고 새로 정의한 getWeather()에 매개변수로 전달하여 날씨를 받아온다.
getWeather에서는 전달받은 latitude, longitude를 이용하여 앞서 설명한 api를 HTTP통신으로 날씨 정보를 얻어 온다.
HTTP 통신이 필요하기 때문에 'axios' 모듈을 설치해야 한다. 설치 명령어는 아래와 같다.
npm install axios
axios.get()을 이용하여 날씨 정보를 얻어 오자.
api.openweathermap.org/data/2.5/weather?lat={lat}&lon={lon}&appid={your api key}
lat에는 latitude를 lon에는 longitude를 올바르게 넣어주면 된다. api key에는 로그인하고 받은 api key를 스트링 형태로 전달하면 된다.
( jsx 문법을 이용하여 매개 변수를 전달해야 하기 때문에 무조건 '백틱'을 사용해야 한다. )
그 뒤, log를 찍은 결과이다.
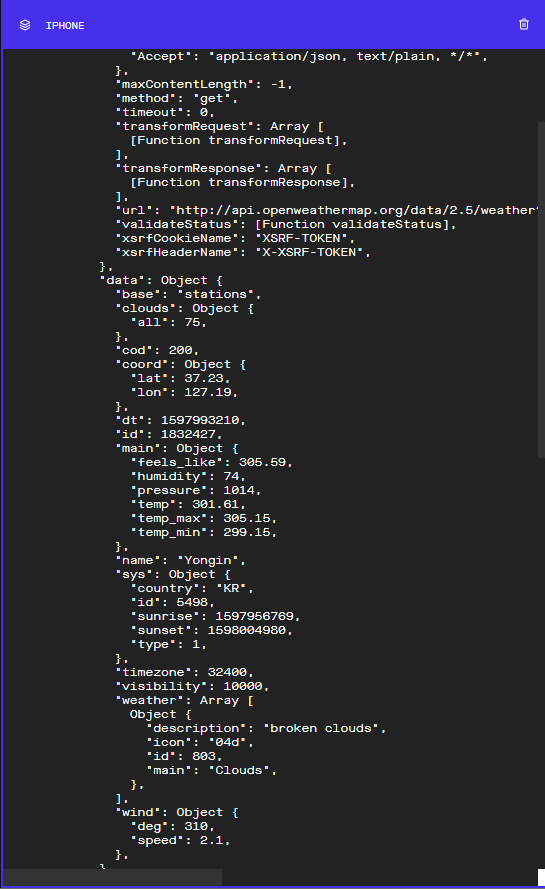
Get Weather
이전 포스트에서 위치를 받아와 위도, 경도를 알아내 log를 찍어보았다.
이제는 위도, 경도를 이용하여 해당 지역에 날씨를 받아오는 일을 하면 프로젝트의 전반적인 부분을 다 마치게 된다.
날씨를 받아오는 방법은 아래의 api를 이용한다.
Сurrent weather and forecast - OpenWeatherMap
Dashboard and Agro API We provide satellite imagery, weather data and other agricultural services that are based on geodata. By using Agro API , you can easily power your solution based on this information. Dashboard is a visual service where you can easy
openweathermap.org
회원 가입을 하면 api key를 부여받을 수 있다.
https://openweathermap.org/current
Current weather data - OpenWeatherMap
Access current weather data for any location on Earth including over 200,000 cities! Current weather is frequently updated based on global models and data from more than 40,000 weather stations. Data is available in JSON, XML, or HTML format. We provide 40
openweathermap.org
이 페이지에 나오는
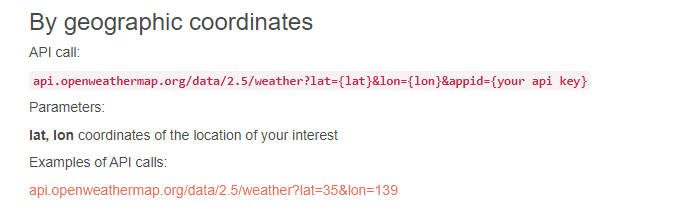
위도, 경도 좌표로 날씨를 받아오는 api를 참고하여 이전 포스트에 받은 위도, 경도를 입력하면 완료된다.
다음은 수정된 App.js이다.
import React from 'react';
import { StyleSheet, Text, View } from 'react-native';
import Loading from './Loading';
import * as Location from 'expo-location';
import * as axios from 'axios';
const API_key = 'e096ae46f45b79ac3723946f3382abb6';
export default class App extends React.Component{
constructor(props){
super(props);
}
state = {
errorMsg: ' ',
isLoading: true,
}
getLocation = async() =>{
const status = await Location.requestPermissionsAsync();
if (status !== 'granted') {
this.setState({errorMsg : 'Permission to access location was denied'});
console.log(this.state.errorMsg);
}
const {
coords: {latitude, longitude}
} = await Location.getCurrentPositionAsync();
this.getWeather(latitude, longitude);
}
getWeather = async(latitude, longitude) => {
const data = await axios.get(
`http://api.openweathermap.org/data/2.5/weather?lat=${latitude}&lon=${longitude}&appid=${API_key}`
);
console.log(data);
}
componentDidMount(){
this.getLocation();
}
render(){
return <Loading/>;
}
}
Location.getCurrentPositionAsync()를 통해서 위치 정보를 얻어왔다 그 얻어온 정보 중, coord라는 객체 안에 들어있는 latitude, longitude를 받아온다.
그리고 새로 정의한 getWeather()에 매개변수로 전달하여 날씨를 받아온다.
getWeather에서는 전달받은 latitude, longitude를 이용하여 앞서 설명한 api를 HTTP통신으로 날씨 정보를 얻어 온다.
HTTP 통신이 필요하기 때문에 'axios' 모듈을 설치해야 한다. 설치 명령어는 아래와 같다.
npm install axios
axios.get()을 이용하여 날씨 정보를 얻어 오자.
api.openweathermap.org/data/2.5/weather?lat={lat}&lon={lon}&appid={your api key}
lat에는 latitude를 lon에는 longitude를 올바르게 넣어주면 된다. api key에는 로그인하고 받은 api key를 스트링 형태로 전달하면 된다.
( jsx 문법을 이용하여 매개 변수를 전달해야 하기 때문에 무조건 '백틱'을 사용해야 한다. )
그 뒤, log를 찍은 결과이다.
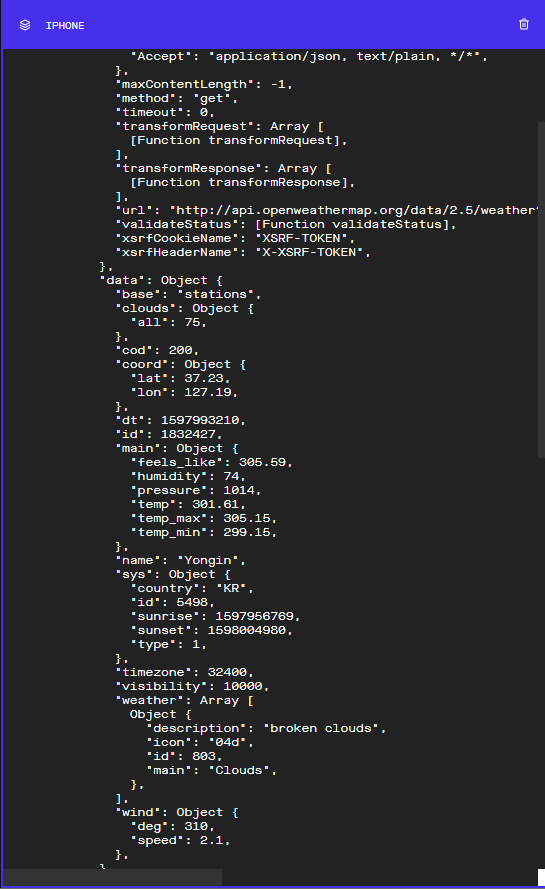