종속성 분석 툴 제작
종속성 분석이 끝난 노드를 보기 좋게 그리는 작업을 해보자.
종속성의 계층 구조 및 흐름을 보기 좋게 트리 형식으로 그리며 사이클이 판정되면 해당 사이클의 연결선은 빨간색으로 표시해 보자.
데이터 저장을 위한 구조 변경
종속성 분석이 완료된 상태이니 노드를 보기 좋게 배치하는 작업이 필요하다.
여러 시도를 해봤지만 선택한 노드를 중앙에 배치하고 방사형으로 퍼져 나가는 그림이 가장 보기 좋았다.
그렇기 때문에 종속성을 depth를 기준으로 표현해야 한다.
struct를 통해 이를 표현해 보자.
public class AssetNode
{
public string Path; // 에셋 경로
public int Depth; // 루트 노드로부터의 깊이
public List<string> Dependencies; // 이 노드가 의존하는 에셋들
public List<AssetNode> Children; // 이 노드의 자식 노드들
public AssetNode Parent; // 부모 노드
public AssetNode(string path)
{
Path = path;
Depth = 0;
Dependencies = new List<string>();
Children = new List<AssetNode>();
Parent = null;
}
// 자식 노드 추가 및 깊이 설정
public void AddChild(AssetNode child)
{
child.Parent = this;
child.Depth = this.Depth + 1;
Children.Add(child);
}
}
에셋의 경로와 선택한 에셋으로부터 참조 거리 등을 저장한다.
해당 자료구조에 맞게 개선된 종속성 분석 흐름은 다음과 같다.
private void CalculateDependency()
{
// 데이터 초기화
assetNodes.Clear();
nodePositions.Clear();
cyclicEdges.Clear();
analysisCompleted = false;
// 루트 노드 생성
AssetNode rootNode = new AssetNode(selectedAsset);
rootNode.Depth = 0;
assetNodes[selectedAsset] = rootNode;
// 처리된 에셋을 추적하기 위한 집합
HashSet<string> processedAssets = new HashSet<string>();
// 재귀적으로 종속성 분석 및 AssetNode 맵 구축
AnalyzeAssetRecursively(selectedAsset, processedAssets, 0, maxAnalysisDepth);
// 순환 참조 감지
DetectCycles();
// 노드 배치
ArrangeNodesWithHierarchy();
analysisCompleted = true;
}
기존 데이터를 초기화 한 후 재귀적으로 종속성을 분석한다.
분석이 완료되면 구조체에 저장된 정보를 기반으로 노드를 배치한다.
순환 참조 탐지
종속성 분석 툴을 만드는 원인인 순환 참조를 탐지하는 로직을 구현해 보자.
private void DetectCycles()
{
cyclicEdges.Clear();
HashSet<string> visited = new HashSet<string>();
HashSet<string> inStack = new HashSet<string>();
// 모든 노드에 대해 순환 참조 검사
foreach (var node in assetNodes.Keys)
{
if (!visited.Contains(node))
{
DFSForCycleDetection(node, visited, inStack);
}
}
}
private void DFSForCycleDetection(string node, HashSet<string> visited, HashSet<string> inStack)
{
visited.Add(node);
inStack.Add(node);
foreach (var dep in assetNodes[node].Dependencies)
{
if (!visited.Contains(dep))
{
DFSForCycleDetection(dep, visited, inStack);
}
else if (inStack.Contains(dep))
{
// 사이클 발견
cyclicEdges.Add(new Tuple<string, string>(node, dep));
// 사이클 관련 노드 표시
if (assetNodes.ContainsKey(node))
assetNodes[node].InCycle = true;
if (assetNodes.ContainsKey(dep))
assetNodes[dep].InCycle = true;
}
}
inStack.Remove(node);
}
모든 노드에 대해 DFS를 진행하여 방문한 노드를 visited에 저장한다.
현재 노드에서 참조하는 에셋들 중 방문하지 않은 노드 대해 DFS를 재귀적으로 진행한다.
만약 방문한 노드이면서 inStack에 있는 노드라면 사이클이 발견된 것이다.
발견한 사이클에 대해 표시를 해준다.
노드 배치
저장된 정보(dpeth)를 통해 노드를 배치해 보자.
// 가상 캔버스 중심점을 기준으로 좌표 계산
float centerX = canvasCenter.x;
float centerY = canvasCenter.y;
// 1. 루트 노드 배치
if (!selectedAsset.IsNullOrEmpty() && assetNodes.ContainsKey(selectedAsset))
{
nodePositions[selectedAsset] = new Rect(
centerX - nodeWidth/2,
centerY - nodeHeight/2,
nodeWidth,
nodeHeight
);
}
캔버스 중앙에 선택한 노드를 배치한다.
이후, depth가 1인 노드(선택한 에셋이 직접 참조하는 에셋)를 360도를 균등하게 나누어 배치한다.
// 2. 깊이 1인 노드들을 360도로 균등 분포 (주요 방향성 설정)
var depth1Nodes = assetNodes.Values
.Where(node => node.Depth == 1)
.ToList();
int totalDirections = Mathf.Max(8, depth1Nodes.Count); // 최소 8방향 보장
// 각 노드가 담당할 방향 영역 할당
Dictionary<string, float> nodeBaseAngles = new Dictionary<string, float>();
for (int i = 0; i < depth1Nodes.Count; i++)
{
AssetNode node = depth1Nodes[i];
// 360도를 균등하게 분할
float angle = (2f * Mathf.PI * i) / totalDirections;
nodeBaseAngles[node.Path] = angle;
// 초기 위치 계산
float radius = 350f; // 1단계 깊이 반지름
float x = centerX + Mathf.Cos(angle) * radius - nodeWidth/2;
float y = centerY + Mathf.Sin(angle) * radius - nodeHeight/2;
// 노드 배치
Rect newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
// 충돌 검사 및 회피
while (IsOverlapping(newRect, occupiedAreas))
{
radius += 50f;
x = centerX + Mathf.Cos(angle) * radius - nodeWidth/2;
y = centerY + Mathf.Sin(angle) * radius - nodeHeight/2;
newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
}
nodePositions[node.Path] = newRect;
occupiedAreas.Add(newRect);
}
노드의 개수에 따라 방향을 설정한 후 x, y좌표를 구해낸다.
이후, 겹치는 노드가 있다면 조정하여 새로운 위치를 정한다.
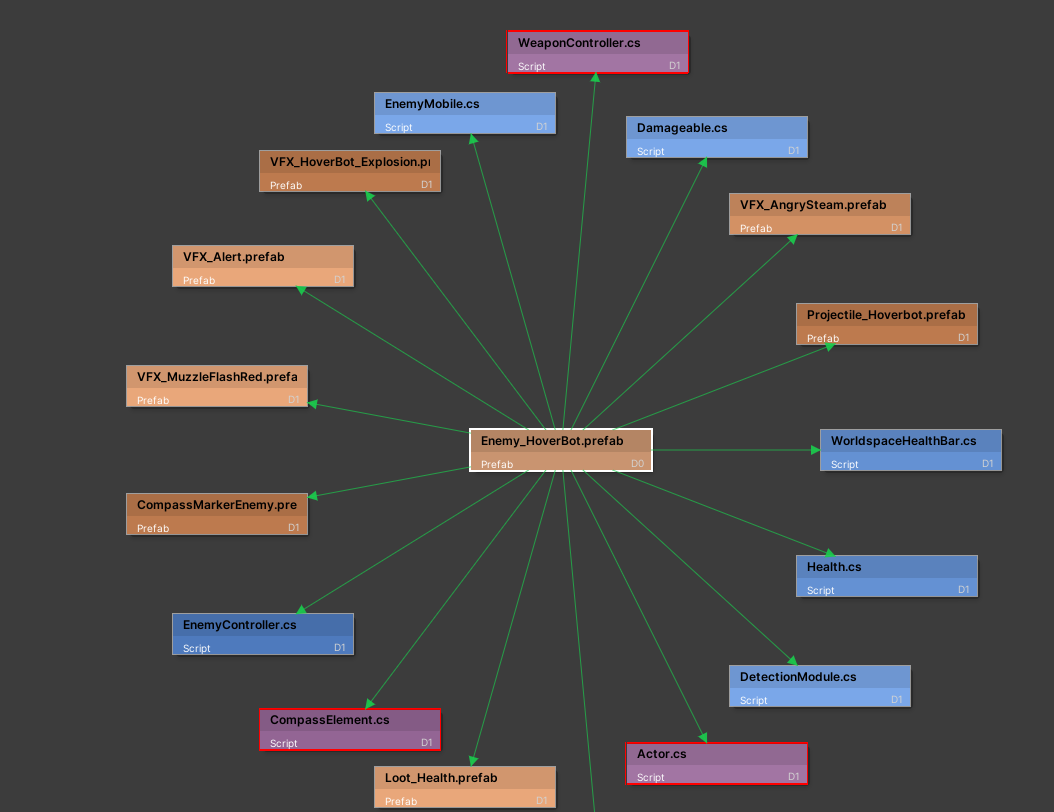
WeaponController의 경우가 겹치는 노드가 있어 조정한 노드이다.
이후 노드들은 자신의 부모가 가지는 방향을 유지하면서 퍼져나가면 된다.
int maxDepth = assetNodes.Values.Any() ? assetNodes.Values.Max(n => n.Depth) : 0;
for (int depth = 2; depth <= maxDepth; depth++)
{
var nodesInDepth = assetNodes.Values
.Where(node => node.Depth == depth)
.ToList();
...
}
최대 깊이를 구하고 depth가 i인 노드들을 Linq를 통해 List로 만들어 배치를 시작한다.
// 부모별로 자식 노드들을 그룹화
var nodesByParent = nodesInDepth.GroupBy(n => n.Parent.Path).ToList();
foreach (var parentGroup in nodesByParent)
{
string parentPath = parentGroup.Key;
var childNodes = parentGroup.ToList();
int childCount = childNodes.Count;
if (!nodePositions.ContainsKey(parentPath)) continue;
AssetNode parentNode = assetNodes[parentPath];
Rect parentRect = nodePositions[parentPath];
Vector2 parentCenter = new Vector2(
parentRect.x + parentRect.width/2,
parentRect.y + parentRect.height/2
);
// 성장 방향 결정 (부모-조부모 방향 사용)
float growthAngle = 0;
if (parentNode.Parent != null && nodePositions.ContainsKey(parentNode.Parent.Path))
{
Rect grandparentRect = nodePositions[parentNode.Parent.Path];
Vector2 grandparentCenter = new Vector2(
grandparentRect.x + grandparentRect.width/2,
grandparentRect.y + grandparentRect.height/2
);
Vector2 growthDir = new Vector2(
parentCenter.x - grandparentCenter.x,
parentCenter.y - grandparentCenter.y
);
if (growthDir.magnitude > 1.0f)
{
growthAngle = Mathf.Atan2(growthDir.y, growthDir.x);
}
}
...
}
부모의 위치가 정해진 경우 최대한 방향성을 유지하도록 계산한다.
같은 depth내의 같은 부모를 갖는 노드를 Linq를 통해 그룹화하여 노드의 위치를 결정한다.
부모와 조부모사이의 벡터를 구한 뒤 Mathf.Atan2를 이용하여 각도를 계산한다.
// 자식 노드 개수에 따른 배치 전략
float baseRadius = 60f + (depth * 120f);
float angleSpread;
// 자식 수에 따라 분산 각도 조정
if (childCount <= 3)
{
angleSpread = Mathf.PI / 6; // 30도 (±15도)
}
else if (childCount <= 6)
{
angleSpread = Mathf.PI / 4; // 45도 (±22.5도)
}
else
{
angleSpread = Mathf.PI / 3; // 60도 (±30도)
}
그리고 자식 노드의 개수에 따라 각도를 조정한다.
// 각 자식 노드 배치
for (int i = 0; i < childCount; i++)
{
AssetNode childNode = childNodes[i];
// 이미 배치된 노드는 건너뛰기
if (nodePositions.ContainsKey(childNode.Path)) continue;
// 자식 위치 계산 (부모로부터 일정 거리, 분산된 각도)
float childAngle = growthAngle;
if (childCount > 1)
{
// -1.0 ~ +1.0 범위로 정규화된 오프셋
float normalizedOffset = (2.0f * i / (childCount - 1)) - 1.0f;
childAngle += angleSpread * normalizedOffset;
}
// 충돌 회피를 위한 위치 시도
bool positionFound = false;
float radius = baseRadius;
int attempts = 0;
while (!positionFound && attempts < 12)
{
float x = parentCenter.x + Mathf.Cos(childAngle) * radius - nodeWidth/2;
float y = parentCenter.y + Mathf.Sin(childAngle) * radius - nodeHeight/2;
Rect newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
if (!IsOverlapping(newRect, occupiedAreas))
{
nodePositions[childNode.Path] = newRect;
occupiedAreas.Add(newRect);
positionFound = true;
}
else
{
attempts++;
// 충돌 회피 전략 수정 - 각도와 거리 모두 조정
if (attempts % 2 == 0)
{
// 거리 증가
radius += 50f;
}
else
{
// 각도 미세 조정 (방향 번갈아가며)
float angleAdjust = (Mathf.PI / 12) * (attempts % 4 == 1 ? 1 : -1);
childAngle += angleAdjust;
}
}
}
// 위치를 찾지 못했다면 강제 배치
if (!positionFound)
{
float x = parentCenter.x + Mathf.Cos(childAngle) * (baseRadius + 300f) - nodeWidth/2;
float y = parentCenter.y + Mathf.Sin(childAngle) * (baseRadius + 300f) - nodeHeight/2;
Rect newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
nodePositions[childNode.Path] = newRect;
occupiedAreas.Add(newRect);
}
}
이후, 실제로 노드 배치를 시도한다.
만약 겹치는 노드가 있다면 각도와 거리를 조금씩 바꿔가며 조정하고 실패한다면 강제로 배치한다.
겹치는 노드를 판단하는 로직은 다음과 같다.
private bool IsOverlapping(Rect rect, HashSet<Rect> existingRects)
{
foreach (var existingRect in existingRects)
{
// 여백을 추가하여 충분한 간격 보장
Rect expandedExisting = new Rect(
existingRect.x - 10,
existingRect.y - 10,
existingRect.width + 20,
existingRect.height + 20
);
if (rect.Overlaps(expandedExisting))
{
return true;
}
}
return false;
}
노드 배치 전체 코드
private void ArrangeNodesWithHierarchy()
{
nodePositions.Clear();
// 가상 캔버스 중심점을 기준으로 좌표 계산
float centerX = canvasCenter.x;
float centerY = canvasCenter.y;
// 1. 루트 노드 배치
if (!selectedAsset.IsNullOrEmpty() && assetNodes.ContainsKey(selectedAsset))
{
nodePositions[selectedAsset] = new Rect(
centerX - nodeWidth/2,
centerY - nodeHeight/2,
nodeWidth,
nodeHeight
);
}
// 충돌 감지 및 방지를 위한 HashSet
HashSet<Rect> occupiedAreas = new HashSet<Rect>();
// 이미 배치된 노드의 영역 추가
foreach (var rect in nodePositions.Values)
{
occupiedAreas.Add(rect);
}
// 2. 깊이 1인 노드들을 360도로 균등 분포 (주요 방향성 설정)
var depth1Nodes = assetNodes.Values
.Where(node => node.Depth == 1)
.ToList();
int totalDirections = Mathf.Max(8, depth1Nodes.Count); // 최소 8방향 보장
// 각 노드가 담당할 방향 영역 할당
Dictionary<string, float> nodeBaseAngles = new Dictionary<string, float>();
for (int i = 0; i < depth1Nodes.Count; i++)
{
AssetNode node = depth1Nodes[i];
// 360도를 균등하게 분할
float angle = (2f * Mathf.PI * i) / totalDirections;
nodeBaseAngles[node.Path] = angle;
// 초기 위치 계산
float radius = 350f; // 1단계 깊이 반지름
float x = centerX + Mathf.Cos(angle) * radius - nodeWidth/2;
float y = centerY + Mathf.Sin(angle) * radius - nodeHeight/2;
// 노드 배치
Rect newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
// 충돌 검사 및 회피
while (IsOverlapping(newRect, occupiedAreas))
{
radius += 50f;
x = centerX + Mathf.Cos(angle) * radius - nodeWidth/2;
y = centerY + Mathf.Sin(angle) * radius - nodeHeight/2;
newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
}
nodePositions[node.Path] = newRect;
occupiedAreas.Add(newRect);
}
// 3. 나머지 깊이의 노드들을 자신의 부모나 참조 노드의 방향으로 분포
int maxDepth = assetNodes.Values.Any() ? assetNodes.Values.Max(n => n.Depth) : 0;
for (int depth = 2; depth <= maxDepth; depth++)
{
var nodesInDepth = assetNodes.Values
.Where(node => node.Depth == depth)
.ToList();
// 부모별로 자식 노드들을 그룹화
var nodesByParent = nodesInDepth.GroupBy(n => n.Parent.Path).ToList();
// 각 부모 노드에 대해 자식들을 분산 배치
foreach (var parentGroup in nodesByParent)
{
string parentPath = parentGroup.Key;
var childNodes = parentGroup.ToList();
int childCount = childNodes.Count;
if (!nodePositions.ContainsKey(parentPath)) continue;
AssetNode parentNode = assetNodes[parentPath];
Rect parentRect = nodePositions[parentPath];
Vector2 parentCenter = new Vector2(
parentRect.x + parentRect.width/2,
parentRect.y + parentRect.height/2
);
// 성장 방향 결정 (부모-조부모 방향 사용)
float growthAngle = 0;
if (parentNode.Parent != null && nodePositions.ContainsKey(parentNode.Parent.Path))
{
Rect grandparentRect = nodePositions[parentNode.Parent.Path];
Vector2 grandparentCenter = new Vector2(
grandparentRect.x + grandparentRect.width/2,
grandparentRect.y + grandparentRect.height/2
);
Vector2 growthDir = new Vector2(
parentCenter.x - grandparentCenter.x,
parentCenter.y - grandparentCenter.y
);
if (growthDir.magnitude > 1.0f)
{
growthAngle = Mathf.Atan2(growthDir.y, growthDir.x);
}
}
// 자식 노드 개수에 따른 배치 전략
float baseRadius = 60f + (depth * 120f);
float angleSpread;
// 자식 수에 따라 분산 각도 조정
if (childCount <= 3)
{
angleSpread = Mathf.PI / 6; // 30도 (±15도)
}
else if (childCount <= 6)
{
angleSpread = Mathf.PI / 4; // 45도 (±22.5도)
}
else
{
angleSpread = Mathf.PI / 3; // 60도 (±30도)
}
// 각 자식 노드 배치
for (int i = 0; i < childCount; i++)
{
AssetNode childNode = childNodes[i];
// 이미 배치된 노드는 건너뛰기
if (nodePositions.ContainsKey(childNode.Path)) continue;
// 자식 위치 계산 (부모로부터 일정 거리, 분산된 각도)
float childAngle = growthAngle;
if (childCount > 1)
{
// -1.0 ~ +1.0 범위로 정규화된 오프셋
float normalizedOffset = (2.0f * i / (childCount - 1)) - 1.0f;
childAngle += angleSpread * normalizedOffset;
}
// 충돌 회피를 위한 위치 시도
bool positionFound = false;
float radius = baseRadius;
int attempts = 0;
while (!positionFound && attempts < 12)
{
float x = parentCenter.x + Mathf.Cos(childAngle) * radius - nodeWidth/2;
float y = parentCenter.y + Mathf.Sin(childAngle) * radius - nodeHeight/2;
Rect newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
if (!IsOverlapping(newRect, occupiedAreas))
{
nodePositions[childNode.Path] = newRect;
occupiedAreas.Add(newRect);
positionFound = true;
}
else
{
attempts++;
// 충돌 회피 전략 수정 - 각도와 거리 모두 조정
if (attempts % 2 == 0)
{
// 거리 증가
radius += 50f;
}
else
{
// 각도 미세 조정 (방향 번갈아가며)
float angleAdjust = (Mathf.PI / 12) * (attempts % 4 == 1 ? 1 : -1);
childAngle += angleAdjust;
}
}
}
// 위치를 찾지 못했다면 강제 배치
if (!positionFound)
{
float x = parentCenter.x + Mathf.Cos(childAngle) * (baseRadius + 300f) - nodeWidth/2;
float y = parentCenter.y + Mathf.Sin(childAngle) * (baseRadius + 300f) - nodeHeight/2;
Rect newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
nodePositions[childNode.Path] = newRect;
occupiedAreas.Add(newRect);
}
}
}
}
// 초기 스크롤 위치 설정
InitializeScrollPosition();
}
노드 + 연결선 그리기
이제 계산된 노드와 노드들 사이에 연결선을 그려보자.
private void DrawNodes()
{
if (Event.current.type != EventType.Repaint)
return;
if (nodePositions.Count == 0)
return;
// 최대 깊이 찾기
int maxDepth = assetNodes.Values.Any() ? assetNodes.Values.Max(n => n.Depth) : 1;
// nodePositions에 있는 모든 키를 순회
foreach (var assetPath in nodePositions.Keys)
{
// 깊이 필터링 적용
if (assetNodes.ContainsKey(assetPath) && assetNodes[assetPath].Depth > maxDepthToShow)
continue;
Rect position = nodePositions[assetPath];
string fileName = System.IO.Path.GetFileName(assetPath);
string fileExt = System.IO.Path.GetExtension(assetPath).ToLower();
// 노드 색상 팔레트 개선
Color[] scriptColors = {
new Color(0.3f, 0.5f, 0.8f), // 파란색 계열
new Color(0.4f, 0.6f, 0.9f),
new Color(0.2f, 0.4f, 0.7f)
};
Color[] prefabColors = {
new Color(0.8f, 0.5f, 0.3f), // 주황색 계열
new Color(0.9f, 0.6f, 0.4f),
new Color(0.7f, 0.4f, 0.2f)
};
Color nodeColor = Color.gray;
string nodeType = "Unknown";
switch (fileExt)
{
case ".cs":
nodeColor = scriptColors[Math.Abs(assetPath.GetHashCode()) % scriptColors.Length];
nodeType = "Script";
break;
case ".prefab":
nodeColor = prefabColors[Math.Abs(assetPath.GetHashCode()) % prefabColors.Length];
nodeType = "Prefab";
break;
case ".asset":
nodeColor = new Color(0.5f, 0.8f, 0.3f); // 연두색 (에셋)
nodeType = "Asset";
break;
}
// 깊이에 따른 색상 조정 (깊이가 깊을수록 밝아짐)
int depth = assetNodes[assetPath].Depth;
float depthRatio = maxDepth > 0 ? (float)depth / maxDepth : 0;
nodeColor = Color.Lerp(nodeColor, Color.white, depthRatio * 0.4f);
// 선택된 에셋 강조 (초기 선택된 에셋 또는 클릭된 에셋)
bool isSelected = selectedAsset.Equals(assetPath);
bool isHighlighted = assetPath == highlightedAsset;
if (isHighlighted)
{
// 하이라이트된 노드는 밝게 강조
nodeColor = Color.Lerp(nodeColor, Color.yellow, 0.5f);
}
else if (isSelected)
{
// 선택된 에셋은 약간 밝게
nodeColor = Color.Lerp(nodeColor, Color.white, 0.3f);
}
// 순환 참조를 포함하는 노드 표시
if (assetNodes[assetPath].InCycle)
{
// 약간 빨간색 추가
nodeColor = Color.Lerp(nodeColor, Color.red, 0.3f);
}
// 노드 그리기
DrawSingleNode(position, fileName, nodeType, nodeColor, isSelected || isHighlighted);
// 깊이 정보 표시 (노드 오른쪽 아래에 작게 표시)
GUI.Label(
new Rect(position.x + position.width - 25, position.y + position.height - 15, 20, 15),
$"D{depth}",
new GUIStyle(EditorStyles.miniLabel) { alignment = TextAnchor.MiddleRight }
);
}
}
미리 계산된 nodePosion을 이용하면 쉽게 노드를 그릴 수 있다.
해당 메서드에서 하는 작업은 depth와 종류에 따라 색상을 구분 지어주며 해당 노드를 클릭했을 때 등 색상과 외형을 설정하는 작업이다.
실제로 노드를 그리는 메서드는 다음과 같다.
private void DrawSingleNode(Rect position, string title, string type, Color color, bool isSelected = false)
{
// 파일 이름만 추출
string displayName = System.IO.Path.GetFileName(title);
bool isInCycle = cyclicEdges != null &&
cyclicEdges.Any(e =>
e.Item1 == title || e.Item2 == title ||
(System.IO.Path.GetFileName(e.Item1) == displayName || System.IO.Path.GetFileName(e.Item2) == displayName));
// 그림자 효과
GUI.color = new Color(0, 0, 0, 0.3f);
GUI.Box(new Rect(position.x + 3, position.y + 3, position.width, position.height), "", "flow node 0");
GUI.color = Color.white;
// 노드 배경
EditorGUI.DrawRect(position, color);
// 테두리 색상 선택
Color borderColor;
float borderWidth;
if (title == highlightedAsset)
{
// 하이라이트된 노드 - 노란색 굵은 테두리
borderColor = Color.yellow;
borderWidth = 3f;
}
else if (isSelected)
{
// 선택된 노드 - 흰색 테두리
borderColor = Color.white;
borderWidth = 2f;
}
else if (isInCycle)
{
// 사이클 포함 노드 - 빨간색 테두리
borderColor = Color.red;
borderWidth = 1.5f;
}
else
{
// 일반 노드 - 반투명 테두리
borderColor = new Color(1, 1, 1, 0.5f);
borderWidth = 1f;
}
// 위/아래 테두리
EditorGUI.DrawRect(new Rect(position.x, position.y - borderWidth, position.width, borderWidth), borderColor);
EditorGUI.DrawRect(new Rect(position.x, position.y + position.height, position.width, borderWidth), borderColor);
// 좌/우 테두리
EditorGUI.DrawRect(new Rect(position.x - borderWidth, position.y - borderWidth, borderWidth, position.height + borderWidth * 2), borderColor);
EditorGUI.DrawRect(new Rect(position.x + position.width, position.y - borderWidth, borderWidth, position.height + borderWidth * 2), borderColor);
// 제목 줄 배경 (약간 어두운 그라데이션)
EditorGUI.DrawRect(new Rect(position.x, position.y, position.width, 22), new Color(0, 0, 0, 0.1f));
// 노드 내용 - 파일 이름과 타입 표시
GUIStyle titleStyle = new GUIStyle(EditorStyles.whiteBoldLabel);
titleStyle.alignment = TextAnchor.MiddleLeft;
GUI.Label(new Rect(position.x + 8, position.y, position.width - 16, 22), displayName, titleStyle);
GUIStyle typeStyle = new GUIStyle(EditorStyles.whiteLabel);
typeStyle.alignment = TextAnchor.MiddleLeft;
typeStyle.fontSize = 10;
GUI.Label(new Rect(position.x + 8, position.y + 24, position.width - 16, 20), type, typeStyle);
}
연결선을 그리는 로직은 다음과 같다.
private void DrawConnectionLines()
{
if (Event.current.type != EventType.Repaint)
return;
Handles.BeginGUI();
// 연결선 스타일 정의
float normalLineWidth = 1.5f;
float highlightLineWidth = 2.0f;
Color hierarchyLineColor = new Color(0.1f, 0.8f, 0.3f, 0.9f); // 녹색 (정방향 계층)
Color reverseLineColor = new Color(0.4f, 0.6f, 1.0f, 0.7f); // 파란색 (역방향)
Color cyclicLineColor = new Color(1.0f, 0.3f, 0.3f, 0.8f); // 빨간색 (사이클)
HashSet<Tuple<string, string>> drawnConnections = new HashSet<Tuple<string, string>>();
// 1. 계층 구조 그리기 (부모 -> 자식, 정방향)
foreach (var node in assetNodes.Values)
{
if (node.Parent == null) continue;
// 깊이 필터링
if (node.Depth > maxDepthToShow) continue;
string childPath = node.Path;
string parentPath = node.Parent.Path;
if (!nodePositions.ContainsKey(childPath) || !nodePositions.ContainsKey(parentPath))
continue;
Rect childRect = nodePositions[childPath];
Rect parentRect = nodePositions[parentPath];
// 연결 포인트 계산
Vector2 childCenter = new Vector2(childRect.x + childRect.width/2, childRect.y + childRect.height/2);
Vector2 parentCenter = new Vector2(parentRect.x + parentRect.width/2, parentRect.y + parentRect.height/2);
Vector2 start = GetConnectionPoint(parentRect, childCenter);
Vector2 end = GetConnectionPoint(childRect, parentCenter);
// 계층 구조는 녹색 실선 직선으로 그리기 (부모 -> 자식 방향)
DrawStraightLine(start, end, hierarchyLineColor, highlightLineWidth);
// 그려진 연결 표시
drawnConnections.Add(new Tuple<string, string>(parentPath, childPath));
}
// 계층 구조만 표시하는 경우 종료
if (showHierarchyOnly)
{
Handles.EndGUI();
return;
}
// 2. 종속성 관계 그리기
if (showDirectDependencies)
{
foreach (var sourceNode in assetNodes.Values)
{
string sourceAsset = sourceNode.Path;
// 깊이 필터링
if (sourceNode.Depth > maxDepthToShow)
continue;
if (!nodePositions.ContainsKey(sourceAsset))
continue;
Rect sourceRect = nodePositions[sourceAsset];
foreach (string targetAsset in sourceNode.Dependencies)
{
// 타겟 노드가 없으면 건너뛰기
if (!assetNodes.ContainsKey(targetAsset))
continue;
AssetNode targetNode = assetNodes[targetAsset];
// 깊이 필터링
if (targetNode.Depth > maxDepthToShow)
continue;
if (sourceAsset == targetAsset || !nodePositions.ContainsKey(targetAsset))
continue;
// 이미 그려진 연결은 건너뛰기
var connectionKey = new Tuple<string, string>(sourceAsset, targetAsset);
if (drawnConnections.Contains(connectionKey))
continue;
drawnConnections.Add(connectionKey);
// 종속성 연결
Rect targetRect = nodePositions[targetAsset];
// 연결 포인트 계산
Vector2 sourceCenter = new Vector2(sourceRect.x + sourceRect.width/2, sourceRect.y + sourceRect.height/2);
Vector2 targetCenter = new Vector2(targetRect.x + targetRect.width/2, targetRect.y + targetRect.height/2);
Vector2 start = GetConnectionPoint(sourceRect, targetCenter);
Vector2 end = GetConnectionPoint(targetRect, sourceCenter);
// 사이클 여부 확인
bool isCyclic = cyclicEdges.Contains(new Tuple<string, string>(sourceAsset, targetAsset));
// 사이클 표시 여부 확인
if (isCyclic && !showCyclicDependencies)
continue;
if (isCyclic)
{
// 1. 사이클: 빨간색 실선 곡선
DrawCurvedLine(start, end, cyclicLineColor, highlightLineWidth, 0.3f, false);
}
else if (sourceNode.Depth >= targetNode.Depth)
{
// 2. 역방향 (자신보다 낮은 깊이를 가리키는 경우): 파란색 점선 곡선
DrawCurvedLine(start, end, reverseLineColor, normalLineWidth, 0.3f, true);
}
else
{
// 3. 정방향 (깊이가 증가하는 방향): 녹색 실선 직선
DrawStraightLine(start, end, hierarchyLineColor, normalLineWidth);
}
}
}
}
Handles.EndGUI();
}
결과 이미지
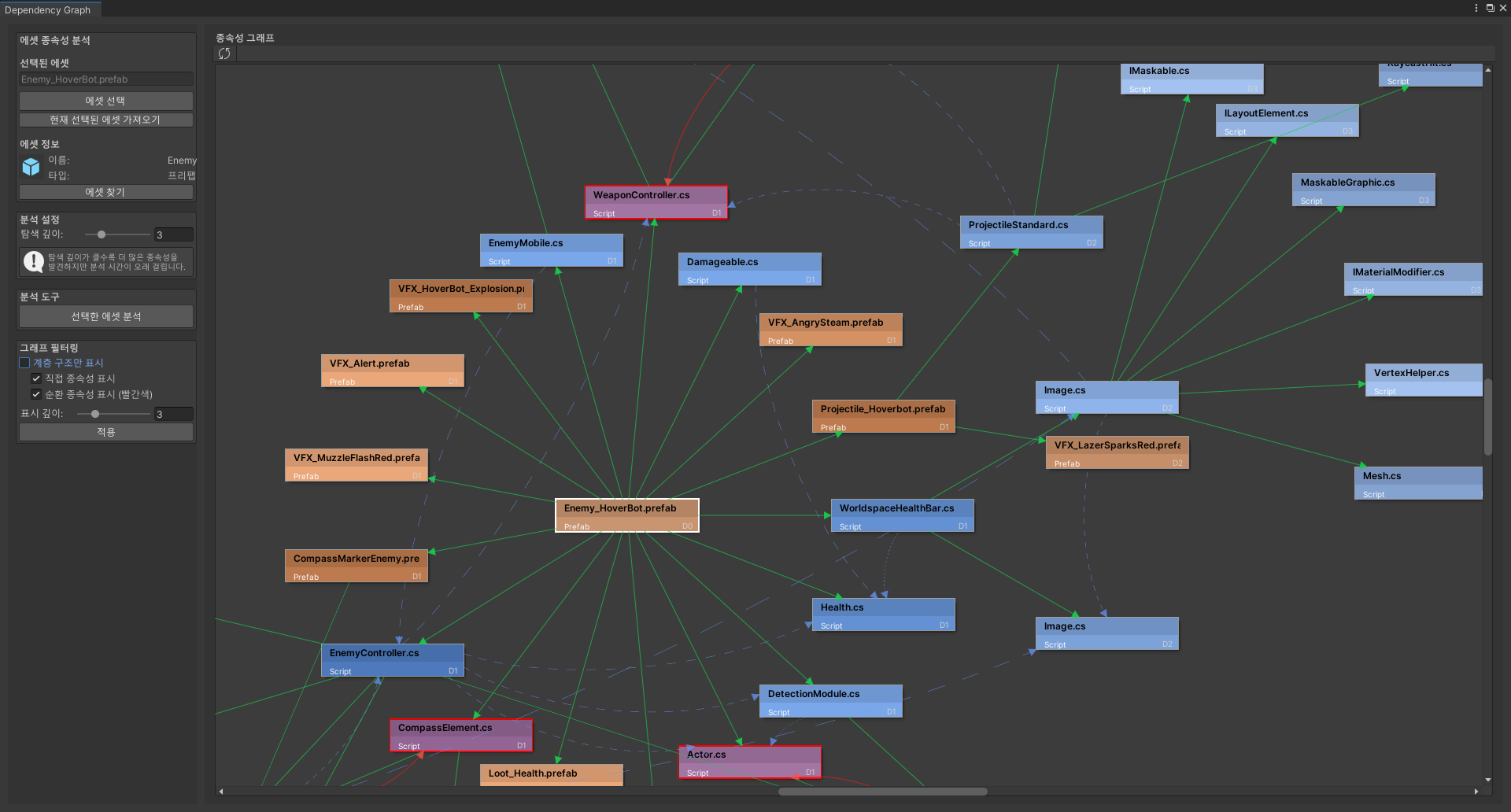
종속성 분석 툴 제작
종속성 분석이 끝난 노드를 보기 좋게 그리는 작업을 해보자.
종속성의 계층 구조 및 흐름을 보기 좋게 트리 형식으로 그리며 사이클이 판정되면 해당 사이클의 연결선은 빨간색으로 표시해 보자.
데이터 저장을 위한 구조 변경
종속성 분석이 완료된 상태이니 노드를 보기 좋게 배치하는 작업이 필요하다.
여러 시도를 해봤지만 선택한 노드를 중앙에 배치하고 방사형으로 퍼져 나가는 그림이 가장 보기 좋았다.
그렇기 때문에 종속성을 depth를 기준으로 표현해야 한다.
struct를 통해 이를 표현해 보자.
public class AssetNode
{
public string Path; // 에셋 경로
public int Depth; // 루트 노드로부터의 깊이
public List<string> Dependencies; // 이 노드가 의존하는 에셋들
public List<AssetNode> Children; // 이 노드의 자식 노드들
public AssetNode Parent; // 부모 노드
public AssetNode(string path)
{
Path = path;
Depth = 0;
Dependencies = new List<string>();
Children = new List<AssetNode>();
Parent = null;
}
// 자식 노드 추가 및 깊이 설정
public void AddChild(AssetNode child)
{
child.Parent = this;
child.Depth = this.Depth + 1;
Children.Add(child);
}
}
에셋의 경로와 선택한 에셋으로부터 참조 거리 등을 저장한다.
해당 자료구조에 맞게 개선된 종속성 분석 흐름은 다음과 같다.
private void CalculateDependency()
{
// 데이터 초기화
assetNodes.Clear();
nodePositions.Clear();
cyclicEdges.Clear();
analysisCompleted = false;
// 루트 노드 생성
AssetNode rootNode = new AssetNode(selectedAsset);
rootNode.Depth = 0;
assetNodes[selectedAsset] = rootNode;
// 처리된 에셋을 추적하기 위한 집합
HashSet<string> processedAssets = new HashSet<string>();
// 재귀적으로 종속성 분석 및 AssetNode 맵 구축
AnalyzeAssetRecursively(selectedAsset, processedAssets, 0, maxAnalysisDepth);
// 순환 참조 감지
DetectCycles();
// 노드 배치
ArrangeNodesWithHierarchy();
analysisCompleted = true;
}
기존 데이터를 초기화 한 후 재귀적으로 종속성을 분석한다.
분석이 완료되면 구조체에 저장된 정보를 기반으로 노드를 배치한다.
순환 참조 탐지
종속성 분석 툴을 만드는 원인인 순환 참조를 탐지하는 로직을 구현해 보자.
private void DetectCycles()
{
cyclicEdges.Clear();
HashSet<string> visited = new HashSet<string>();
HashSet<string> inStack = new HashSet<string>();
// 모든 노드에 대해 순환 참조 검사
foreach (var node in assetNodes.Keys)
{
if (!visited.Contains(node))
{
DFSForCycleDetection(node, visited, inStack);
}
}
}
private void DFSForCycleDetection(string node, HashSet<string> visited, HashSet<string> inStack)
{
visited.Add(node);
inStack.Add(node);
foreach (var dep in assetNodes[node].Dependencies)
{
if (!visited.Contains(dep))
{
DFSForCycleDetection(dep, visited, inStack);
}
else if (inStack.Contains(dep))
{
// 사이클 발견
cyclicEdges.Add(new Tuple<string, string>(node, dep));
// 사이클 관련 노드 표시
if (assetNodes.ContainsKey(node))
assetNodes[node].InCycle = true;
if (assetNodes.ContainsKey(dep))
assetNodes[dep].InCycle = true;
}
}
inStack.Remove(node);
}
모든 노드에 대해 DFS를 진행하여 방문한 노드를 visited에 저장한다.
현재 노드에서 참조하는 에셋들 중 방문하지 않은 노드 대해 DFS를 재귀적으로 진행한다.
만약 방문한 노드이면서 inStack에 있는 노드라면 사이클이 발견된 것이다.
발견한 사이클에 대해 표시를 해준다.
노드 배치
저장된 정보(dpeth)를 통해 노드를 배치해 보자.
// 가상 캔버스 중심점을 기준으로 좌표 계산
float centerX = canvasCenter.x;
float centerY = canvasCenter.y;
// 1. 루트 노드 배치
if (!selectedAsset.IsNullOrEmpty() && assetNodes.ContainsKey(selectedAsset))
{
nodePositions[selectedAsset] = new Rect(
centerX - nodeWidth/2,
centerY - nodeHeight/2,
nodeWidth,
nodeHeight
);
}
캔버스 중앙에 선택한 노드를 배치한다.
이후, depth가 1인 노드(선택한 에셋이 직접 참조하는 에셋)를 360도를 균등하게 나누어 배치한다.
// 2. 깊이 1인 노드들을 360도로 균등 분포 (주요 방향성 설정)
var depth1Nodes = assetNodes.Values
.Where(node => node.Depth == 1)
.ToList();
int totalDirections = Mathf.Max(8, depth1Nodes.Count); // 최소 8방향 보장
// 각 노드가 담당할 방향 영역 할당
Dictionary<string, float> nodeBaseAngles = new Dictionary<string, float>();
for (int i = 0; i < depth1Nodes.Count; i++)
{
AssetNode node = depth1Nodes[i];
// 360도를 균등하게 분할
float angle = (2f * Mathf.PI * i) / totalDirections;
nodeBaseAngles[node.Path] = angle;
// 초기 위치 계산
float radius = 350f; // 1단계 깊이 반지름
float x = centerX + Mathf.Cos(angle) * radius - nodeWidth/2;
float y = centerY + Mathf.Sin(angle) * radius - nodeHeight/2;
// 노드 배치
Rect newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
// 충돌 검사 및 회피
while (IsOverlapping(newRect, occupiedAreas))
{
radius += 50f;
x = centerX + Mathf.Cos(angle) * radius - nodeWidth/2;
y = centerY + Mathf.Sin(angle) * radius - nodeHeight/2;
newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
}
nodePositions[node.Path] = newRect;
occupiedAreas.Add(newRect);
}
노드의 개수에 따라 방향을 설정한 후 x, y좌표를 구해낸다.
이후, 겹치는 노드가 있다면 조정하여 새로운 위치를 정한다.
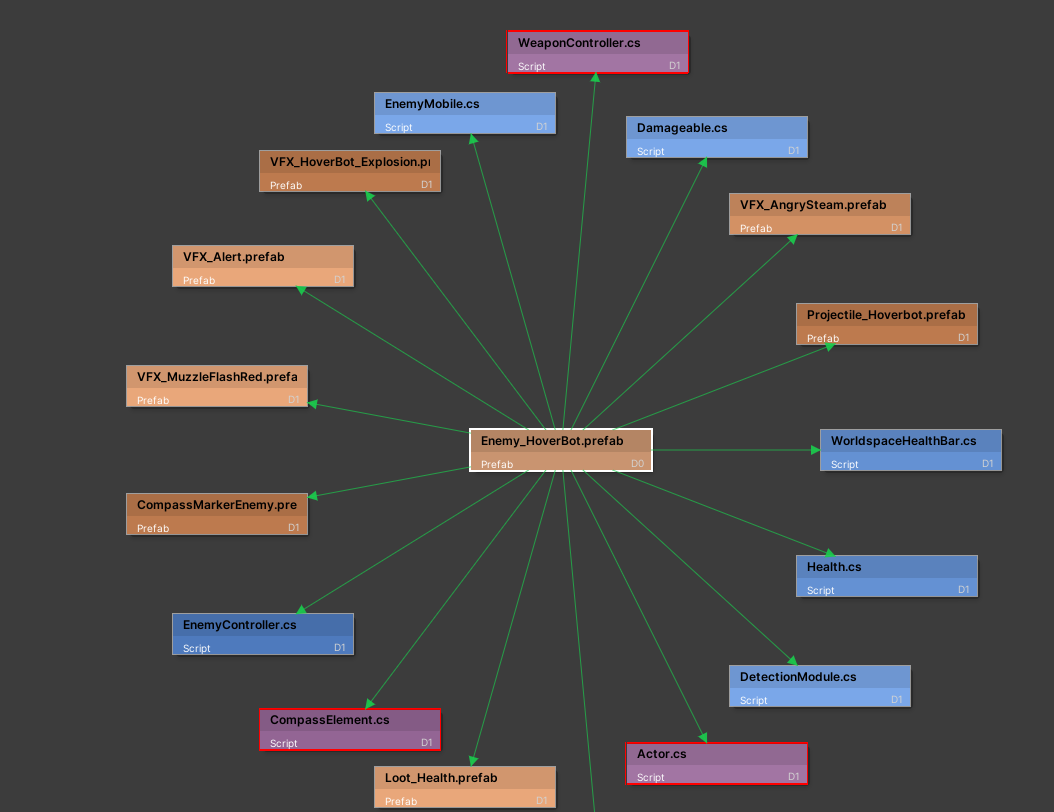
WeaponController의 경우가 겹치는 노드가 있어 조정한 노드이다.
이후 노드들은 자신의 부모가 가지는 방향을 유지하면서 퍼져나가면 된다.
int maxDepth = assetNodes.Values.Any() ? assetNodes.Values.Max(n => n.Depth) : 0;
for (int depth = 2; depth <= maxDepth; depth++)
{
var nodesInDepth = assetNodes.Values
.Where(node => node.Depth == depth)
.ToList();
...
}
최대 깊이를 구하고 depth가 i인 노드들을 Linq를 통해 List로 만들어 배치를 시작한다.
// 부모별로 자식 노드들을 그룹화
var nodesByParent = nodesInDepth.GroupBy(n => n.Parent.Path).ToList();
foreach (var parentGroup in nodesByParent)
{
string parentPath = parentGroup.Key;
var childNodes = parentGroup.ToList();
int childCount = childNodes.Count;
if (!nodePositions.ContainsKey(parentPath)) continue;
AssetNode parentNode = assetNodes[parentPath];
Rect parentRect = nodePositions[parentPath];
Vector2 parentCenter = new Vector2(
parentRect.x + parentRect.width/2,
parentRect.y + parentRect.height/2
);
// 성장 방향 결정 (부모-조부모 방향 사용)
float growthAngle = 0;
if (parentNode.Parent != null && nodePositions.ContainsKey(parentNode.Parent.Path))
{
Rect grandparentRect = nodePositions[parentNode.Parent.Path];
Vector2 grandparentCenter = new Vector2(
grandparentRect.x + grandparentRect.width/2,
grandparentRect.y + grandparentRect.height/2
);
Vector2 growthDir = new Vector2(
parentCenter.x - grandparentCenter.x,
parentCenter.y - grandparentCenter.y
);
if (growthDir.magnitude > 1.0f)
{
growthAngle = Mathf.Atan2(growthDir.y, growthDir.x);
}
}
...
}
부모의 위치가 정해진 경우 최대한 방향성을 유지하도록 계산한다.
같은 depth내의 같은 부모를 갖는 노드를 Linq를 통해 그룹화하여 노드의 위치를 결정한다.
부모와 조부모사이의 벡터를 구한 뒤 Mathf.Atan2를 이용하여 각도를 계산한다.
// 자식 노드 개수에 따른 배치 전략
float baseRadius = 60f + (depth * 120f);
float angleSpread;
// 자식 수에 따라 분산 각도 조정
if (childCount <= 3)
{
angleSpread = Mathf.PI / 6; // 30도 (±15도)
}
else if (childCount <= 6)
{
angleSpread = Mathf.PI / 4; // 45도 (±22.5도)
}
else
{
angleSpread = Mathf.PI / 3; // 60도 (±30도)
}
그리고 자식 노드의 개수에 따라 각도를 조정한다.
// 각 자식 노드 배치
for (int i = 0; i < childCount; i++)
{
AssetNode childNode = childNodes[i];
// 이미 배치된 노드는 건너뛰기
if (nodePositions.ContainsKey(childNode.Path)) continue;
// 자식 위치 계산 (부모로부터 일정 거리, 분산된 각도)
float childAngle = growthAngle;
if (childCount > 1)
{
// -1.0 ~ +1.0 범위로 정규화된 오프셋
float normalizedOffset = (2.0f * i / (childCount - 1)) - 1.0f;
childAngle += angleSpread * normalizedOffset;
}
// 충돌 회피를 위한 위치 시도
bool positionFound = false;
float radius = baseRadius;
int attempts = 0;
while (!positionFound && attempts < 12)
{
float x = parentCenter.x + Mathf.Cos(childAngle) * radius - nodeWidth/2;
float y = parentCenter.y + Mathf.Sin(childAngle) * radius - nodeHeight/2;
Rect newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
if (!IsOverlapping(newRect, occupiedAreas))
{
nodePositions[childNode.Path] = newRect;
occupiedAreas.Add(newRect);
positionFound = true;
}
else
{
attempts++;
// 충돌 회피 전략 수정 - 각도와 거리 모두 조정
if (attempts % 2 == 0)
{
// 거리 증가
radius += 50f;
}
else
{
// 각도 미세 조정 (방향 번갈아가며)
float angleAdjust = (Mathf.PI / 12) * (attempts % 4 == 1 ? 1 : -1);
childAngle += angleAdjust;
}
}
}
// 위치를 찾지 못했다면 강제 배치
if (!positionFound)
{
float x = parentCenter.x + Mathf.Cos(childAngle) * (baseRadius + 300f) - nodeWidth/2;
float y = parentCenter.y + Mathf.Sin(childAngle) * (baseRadius + 300f) - nodeHeight/2;
Rect newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
nodePositions[childNode.Path] = newRect;
occupiedAreas.Add(newRect);
}
}
이후, 실제로 노드 배치를 시도한다.
만약 겹치는 노드가 있다면 각도와 거리를 조금씩 바꿔가며 조정하고 실패한다면 강제로 배치한다.
겹치는 노드를 판단하는 로직은 다음과 같다.
private bool IsOverlapping(Rect rect, HashSet<Rect> existingRects)
{
foreach (var existingRect in existingRects)
{
// 여백을 추가하여 충분한 간격 보장
Rect expandedExisting = new Rect(
existingRect.x - 10,
existingRect.y - 10,
existingRect.width + 20,
existingRect.height + 20
);
if (rect.Overlaps(expandedExisting))
{
return true;
}
}
return false;
}
노드 배치 전체 코드
private void ArrangeNodesWithHierarchy()
{
nodePositions.Clear();
// 가상 캔버스 중심점을 기준으로 좌표 계산
float centerX = canvasCenter.x;
float centerY = canvasCenter.y;
// 1. 루트 노드 배치
if (!selectedAsset.IsNullOrEmpty() && assetNodes.ContainsKey(selectedAsset))
{
nodePositions[selectedAsset] = new Rect(
centerX - nodeWidth/2,
centerY - nodeHeight/2,
nodeWidth,
nodeHeight
);
}
// 충돌 감지 및 방지를 위한 HashSet
HashSet<Rect> occupiedAreas = new HashSet<Rect>();
// 이미 배치된 노드의 영역 추가
foreach (var rect in nodePositions.Values)
{
occupiedAreas.Add(rect);
}
// 2. 깊이 1인 노드들을 360도로 균등 분포 (주요 방향성 설정)
var depth1Nodes = assetNodes.Values
.Where(node => node.Depth == 1)
.ToList();
int totalDirections = Mathf.Max(8, depth1Nodes.Count); // 최소 8방향 보장
// 각 노드가 담당할 방향 영역 할당
Dictionary<string, float> nodeBaseAngles = new Dictionary<string, float>();
for (int i = 0; i < depth1Nodes.Count; i++)
{
AssetNode node = depth1Nodes[i];
// 360도를 균등하게 분할
float angle = (2f * Mathf.PI * i) / totalDirections;
nodeBaseAngles[node.Path] = angle;
// 초기 위치 계산
float radius = 350f; // 1단계 깊이 반지름
float x = centerX + Mathf.Cos(angle) * radius - nodeWidth/2;
float y = centerY + Mathf.Sin(angle) * radius - nodeHeight/2;
// 노드 배치
Rect newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
// 충돌 검사 및 회피
while (IsOverlapping(newRect, occupiedAreas))
{
radius += 50f;
x = centerX + Mathf.Cos(angle) * radius - nodeWidth/2;
y = centerY + Mathf.Sin(angle) * radius - nodeHeight/2;
newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
}
nodePositions[node.Path] = newRect;
occupiedAreas.Add(newRect);
}
// 3. 나머지 깊이의 노드들을 자신의 부모나 참조 노드의 방향으로 분포
int maxDepth = assetNodes.Values.Any() ? assetNodes.Values.Max(n => n.Depth) : 0;
for (int depth = 2; depth <= maxDepth; depth++)
{
var nodesInDepth = assetNodes.Values
.Where(node => node.Depth == depth)
.ToList();
// 부모별로 자식 노드들을 그룹화
var nodesByParent = nodesInDepth.GroupBy(n => n.Parent.Path).ToList();
// 각 부모 노드에 대해 자식들을 분산 배치
foreach (var parentGroup in nodesByParent)
{
string parentPath = parentGroup.Key;
var childNodes = parentGroup.ToList();
int childCount = childNodes.Count;
if (!nodePositions.ContainsKey(parentPath)) continue;
AssetNode parentNode = assetNodes[parentPath];
Rect parentRect = nodePositions[parentPath];
Vector2 parentCenter = new Vector2(
parentRect.x + parentRect.width/2,
parentRect.y + parentRect.height/2
);
// 성장 방향 결정 (부모-조부모 방향 사용)
float growthAngle = 0;
if (parentNode.Parent != null && nodePositions.ContainsKey(parentNode.Parent.Path))
{
Rect grandparentRect = nodePositions[parentNode.Parent.Path];
Vector2 grandparentCenter = new Vector2(
grandparentRect.x + grandparentRect.width/2,
grandparentRect.y + grandparentRect.height/2
);
Vector2 growthDir = new Vector2(
parentCenter.x - grandparentCenter.x,
parentCenter.y - grandparentCenter.y
);
if (growthDir.magnitude > 1.0f)
{
growthAngle = Mathf.Atan2(growthDir.y, growthDir.x);
}
}
// 자식 노드 개수에 따른 배치 전략
float baseRadius = 60f + (depth * 120f);
float angleSpread;
// 자식 수에 따라 분산 각도 조정
if (childCount <= 3)
{
angleSpread = Mathf.PI / 6; // 30도 (±15도)
}
else if (childCount <= 6)
{
angleSpread = Mathf.PI / 4; // 45도 (±22.5도)
}
else
{
angleSpread = Mathf.PI / 3; // 60도 (±30도)
}
// 각 자식 노드 배치
for (int i = 0; i < childCount; i++)
{
AssetNode childNode = childNodes[i];
// 이미 배치된 노드는 건너뛰기
if (nodePositions.ContainsKey(childNode.Path)) continue;
// 자식 위치 계산 (부모로부터 일정 거리, 분산된 각도)
float childAngle = growthAngle;
if (childCount > 1)
{
// -1.0 ~ +1.0 범위로 정규화된 오프셋
float normalizedOffset = (2.0f * i / (childCount - 1)) - 1.0f;
childAngle += angleSpread * normalizedOffset;
}
// 충돌 회피를 위한 위치 시도
bool positionFound = false;
float radius = baseRadius;
int attempts = 0;
while (!positionFound && attempts < 12)
{
float x = parentCenter.x + Mathf.Cos(childAngle) * radius - nodeWidth/2;
float y = parentCenter.y + Mathf.Sin(childAngle) * radius - nodeHeight/2;
Rect newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
if (!IsOverlapping(newRect, occupiedAreas))
{
nodePositions[childNode.Path] = newRect;
occupiedAreas.Add(newRect);
positionFound = true;
}
else
{
attempts++;
// 충돌 회피 전략 수정 - 각도와 거리 모두 조정
if (attempts % 2 == 0)
{
// 거리 증가
radius += 50f;
}
else
{
// 각도 미세 조정 (방향 번갈아가며)
float angleAdjust = (Mathf.PI / 12) * (attempts % 4 == 1 ? 1 : -1);
childAngle += angleAdjust;
}
}
}
// 위치를 찾지 못했다면 강제 배치
if (!positionFound)
{
float x = parentCenter.x + Mathf.Cos(childAngle) * (baseRadius + 300f) - nodeWidth/2;
float y = parentCenter.y + Mathf.Sin(childAngle) * (baseRadius + 300f) - nodeHeight/2;
Rect newRect = new Rect(
Mathf.Max(0, x),
Mathf.Max(0, y),
nodeWidth,
nodeHeight
);
nodePositions[childNode.Path] = newRect;
occupiedAreas.Add(newRect);
}
}
}
}
// 초기 스크롤 위치 설정
InitializeScrollPosition();
}
노드 + 연결선 그리기
이제 계산된 노드와 노드들 사이에 연결선을 그려보자.
private void DrawNodes()
{
if (Event.current.type != EventType.Repaint)
return;
if (nodePositions.Count == 0)
return;
// 최대 깊이 찾기
int maxDepth = assetNodes.Values.Any() ? assetNodes.Values.Max(n => n.Depth) : 1;
// nodePositions에 있는 모든 키를 순회
foreach (var assetPath in nodePositions.Keys)
{
// 깊이 필터링 적용
if (assetNodes.ContainsKey(assetPath) && assetNodes[assetPath].Depth > maxDepthToShow)
continue;
Rect position = nodePositions[assetPath];
string fileName = System.IO.Path.GetFileName(assetPath);
string fileExt = System.IO.Path.GetExtension(assetPath).ToLower();
// 노드 색상 팔레트 개선
Color[] scriptColors = {
new Color(0.3f, 0.5f, 0.8f), // 파란색 계열
new Color(0.4f, 0.6f, 0.9f),
new Color(0.2f, 0.4f, 0.7f)
};
Color[] prefabColors = {
new Color(0.8f, 0.5f, 0.3f), // 주황색 계열
new Color(0.9f, 0.6f, 0.4f),
new Color(0.7f, 0.4f, 0.2f)
};
Color nodeColor = Color.gray;
string nodeType = "Unknown";
switch (fileExt)
{
case ".cs":
nodeColor = scriptColors[Math.Abs(assetPath.GetHashCode()) % scriptColors.Length];
nodeType = "Script";
break;
case ".prefab":
nodeColor = prefabColors[Math.Abs(assetPath.GetHashCode()) % prefabColors.Length];
nodeType = "Prefab";
break;
case ".asset":
nodeColor = new Color(0.5f, 0.8f, 0.3f); // 연두색 (에셋)
nodeType = "Asset";
break;
}
// 깊이에 따른 색상 조정 (깊이가 깊을수록 밝아짐)
int depth = assetNodes[assetPath].Depth;
float depthRatio = maxDepth > 0 ? (float)depth / maxDepth : 0;
nodeColor = Color.Lerp(nodeColor, Color.white, depthRatio * 0.4f);
// 선택된 에셋 강조 (초기 선택된 에셋 또는 클릭된 에셋)
bool isSelected = selectedAsset.Equals(assetPath);
bool isHighlighted = assetPath == highlightedAsset;
if (isHighlighted)
{
// 하이라이트된 노드는 밝게 강조
nodeColor = Color.Lerp(nodeColor, Color.yellow, 0.5f);
}
else if (isSelected)
{
// 선택된 에셋은 약간 밝게
nodeColor = Color.Lerp(nodeColor, Color.white, 0.3f);
}
// 순환 참조를 포함하는 노드 표시
if (assetNodes[assetPath].InCycle)
{
// 약간 빨간색 추가
nodeColor = Color.Lerp(nodeColor, Color.red, 0.3f);
}
// 노드 그리기
DrawSingleNode(position, fileName, nodeType, nodeColor, isSelected || isHighlighted);
// 깊이 정보 표시 (노드 오른쪽 아래에 작게 표시)
GUI.Label(
new Rect(position.x + position.width - 25, position.y + position.height - 15, 20, 15),
$"D{depth}",
new GUIStyle(EditorStyles.miniLabel) { alignment = TextAnchor.MiddleRight }
);
}
}
미리 계산된 nodePosion을 이용하면 쉽게 노드를 그릴 수 있다.
해당 메서드에서 하는 작업은 depth와 종류에 따라 색상을 구분 지어주며 해당 노드를 클릭했을 때 등 색상과 외형을 설정하는 작업이다.
실제로 노드를 그리는 메서드는 다음과 같다.
private void DrawSingleNode(Rect position, string title, string type, Color color, bool isSelected = false)
{
// 파일 이름만 추출
string displayName = System.IO.Path.GetFileName(title);
bool isInCycle = cyclicEdges != null &&
cyclicEdges.Any(e =>
e.Item1 == title || e.Item2 == title ||
(System.IO.Path.GetFileName(e.Item1) == displayName || System.IO.Path.GetFileName(e.Item2) == displayName));
// 그림자 효과
GUI.color = new Color(0, 0, 0, 0.3f);
GUI.Box(new Rect(position.x + 3, position.y + 3, position.width, position.height), "", "flow node 0");
GUI.color = Color.white;
// 노드 배경
EditorGUI.DrawRect(position, color);
// 테두리 색상 선택
Color borderColor;
float borderWidth;
if (title == highlightedAsset)
{
// 하이라이트된 노드 - 노란색 굵은 테두리
borderColor = Color.yellow;
borderWidth = 3f;
}
else if (isSelected)
{
// 선택된 노드 - 흰색 테두리
borderColor = Color.white;
borderWidth = 2f;
}
else if (isInCycle)
{
// 사이클 포함 노드 - 빨간색 테두리
borderColor = Color.red;
borderWidth = 1.5f;
}
else
{
// 일반 노드 - 반투명 테두리
borderColor = new Color(1, 1, 1, 0.5f);
borderWidth = 1f;
}
// 위/아래 테두리
EditorGUI.DrawRect(new Rect(position.x, position.y - borderWidth, position.width, borderWidth), borderColor);
EditorGUI.DrawRect(new Rect(position.x, position.y + position.height, position.width, borderWidth), borderColor);
// 좌/우 테두리
EditorGUI.DrawRect(new Rect(position.x - borderWidth, position.y - borderWidth, borderWidth, position.height + borderWidth * 2), borderColor);
EditorGUI.DrawRect(new Rect(position.x + position.width, position.y - borderWidth, borderWidth, position.height + borderWidth * 2), borderColor);
// 제목 줄 배경 (약간 어두운 그라데이션)
EditorGUI.DrawRect(new Rect(position.x, position.y, position.width, 22), new Color(0, 0, 0, 0.1f));
// 노드 내용 - 파일 이름과 타입 표시
GUIStyle titleStyle = new GUIStyle(EditorStyles.whiteBoldLabel);
titleStyle.alignment = TextAnchor.MiddleLeft;
GUI.Label(new Rect(position.x + 8, position.y, position.width - 16, 22), displayName, titleStyle);
GUIStyle typeStyle = new GUIStyle(EditorStyles.whiteLabel);
typeStyle.alignment = TextAnchor.MiddleLeft;
typeStyle.fontSize = 10;
GUI.Label(new Rect(position.x + 8, position.y + 24, position.width - 16, 20), type, typeStyle);
}
연결선을 그리는 로직은 다음과 같다.
private void DrawConnectionLines()
{
if (Event.current.type != EventType.Repaint)
return;
Handles.BeginGUI();
// 연결선 스타일 정의
float normalLineWidth = 1.5f;
float highlightLineWidth = 2.0f;
Color hierarchyLineColor = new Color(0.1f, 0.8f, 0.3f, 0.9f); // 녹색 (정방향 계층)
Color reverseLineColor = new Color(0.4f, 0.6f, 1.0f, 0.7f); // 파란색 (역방향)
Color cyclicLineColor = new Color(1.0f, 0.3f, 0.3f, 0.8f); // 빨간색 (사이클)
HashSet<Tuple<string, string>> drawnConnections = new HashSet<Tuple<string, string>>();
// 1. 계층 구조 그리기 (부모 -> 자식, 정방향)
foreach (var node in assetNodes.Values)
{
if (node.Parent == null) continue;
// 깊이 필터링
if (node.Depth > maxDepthToShow) continue;
string childPath = node.Path;
string parentPath = node.Parent.Path;
if (!nodePositions.ContainsKey(childPath) || !nodePositions.ContainsKey(parentPath))
continue;
Rect childRect = nodePositions[childPath];
Rect parentRect = nodePositions[parentPath];
// 연결 포인트 계산
Vector2 childCenter = new Vector2(childRect.x + childRect.width/2, childRect.y + childRect.height/2);
Vector2 parentCenter = new Vector2(parentRect.x + parentRect.width/2, parentRect.y + parentRect.height/2);
Vector2 start = GetConnectionPoint(parentRect, childCenter);
Vector2 end = GetConnectionPoint(childRect, parentCenter);
// 계층 구조는 녹색 실선 직선으로 그리기 (부모 -> 자식 방향)
DrawStraightLine(start, end, hierarchyLineColor, highlightLineWidth);
// 그려진 연결 표시
drawnConnections.Add(new Tuple<string, string>(parentPath, childPath));
}
// 계층 구조만 표시하는 경우 종료
if (showHierarchyOnly)
{
Handles.EndGUI();
return;
}
// 2. 종속성 관계 그리기
if (showDirectDependencies)
{
foreach (var sourceNode in assetNodes.Values)
{
string sourceAsset = sourceNode.Path;
// 깊이 필터링
if (sourceNode.Depth > maxDepthToShow)
continue;
if (!nodePositions.ContainsKey(sourceAsset))
continue;
Rect sourceRect = nodePositions[sourceAsset];
foreach (string targetAsset in sourceNode.Dependencies)
{
// 타겟 노드가 없으면 건너뛰기
if (!assetNodes.ContainsKey(targetAsset))
continue;
AssetNode targetNode = assetNodes[targetAsset];
// 깊이 필터링
if (targetNode.Depth > maxDepthToShow)
continue;
if (sourceAsset == targetAsset || !nodePositions.ContainsKey(targetAsset))
continue;
// 이미 그려진 연결은 건너뛰기
var connectionKey = new Tuple<string, string>(sourceAsset, targetAsset);
if (drawnConnections.Contains(connectionKey))
continue;
drawnConnections.Add(connectionKey);
// 종속성 연결
Rect targetRect = nodePositions[targetAsset];
// 연결 포인트 계산
Vector2 sourceCenter = new Vector2(sourceRect.x + sourceRect.width/2, sourceRect.y + sourceRect.height/2);
Vector2 targetCenter = new Vector2(targetRect.x + targetRect.width/2, targetRect.y + targetRect.height/2);
Vector2 start = GetConnectionPoint(sourceRect, targetCenter);
Vector2 end = GetConnectionPoint(targetRect, sourceCenter);
// 사이클 여부 확인
bool isCyclic = cyclicEdges.Contains(new Tuple<string, string>(sourceAsset, targetAsset));
// 사이클 표시 여부 확인
if (isCyclic && !showCyclicDependencies)
continue;
if (isCyclic)
{
// 1. 사이클: 빨간색 실선 곡선
DrawCurvedLine(start, end, cyclicLineColor, highlightLineWidth, 0.3f, false);
}
else if (sourceNode.Depth >= targetNode.Depth)
{
// 2. 역방향 (자신보다 낮은 깊이를 가리키는 경우): 파란색 점선 곡선
DrawCurvedLine(start, end, reverseLineColor, normalLineWidth, 0.3f, true);
}
else
{
// 3. 정방향 (깊이가 증가하는 방향): 녹색 실선 직선
DrawStraightLine(start, end, hierarchyLineColor, normalLineWidth);
}
}
}
}
Handles.EndGUI();
}
결과 이미지
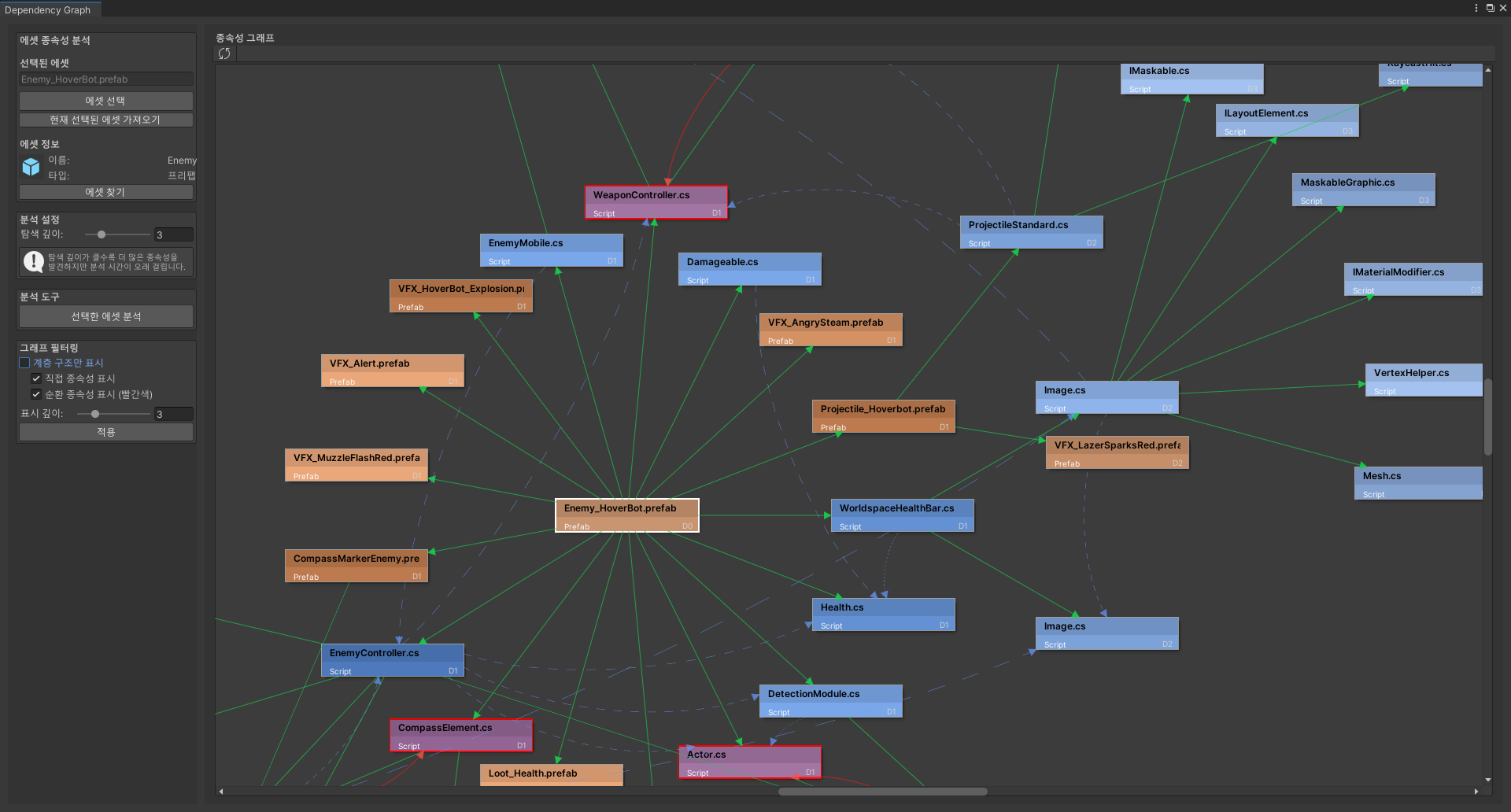